I have been working in React for a long time and have noticed various mistakes made by many developers, including me. Getting into code discussions and code review meetings, I had a chance to know and learn the mistakes I have been making while developing a website in React. I am glad to discuss a few mistakes and how to fix them.
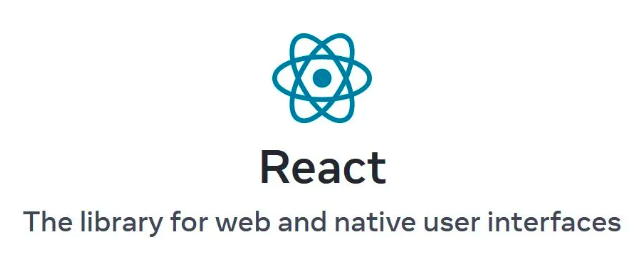
1. Writing many components in a single file
This is one of the most common mistakes that many developer make. Developers tend to write most of the React code in a single file even though they splits the components, still writing all the code in a single file is not the best practice.
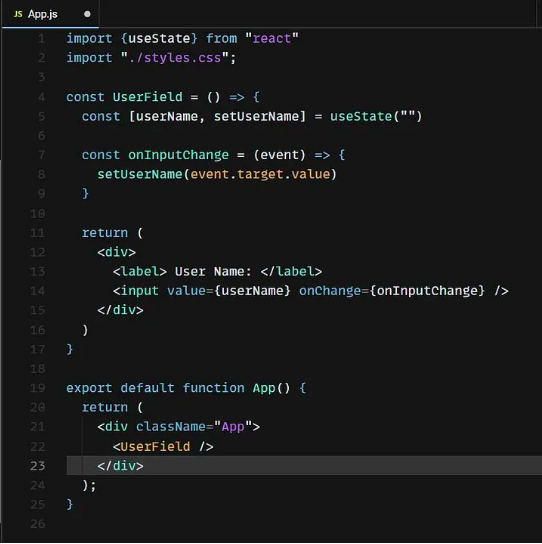
Mistake 1: Writing different component in same file
In the above example, the code will be executed completely fine though the reasons why it is not considered as the best practice are:
- The size of the component will increase by may be 15, 20 or even 30+ lines making the component difficult to understand.
- The
UserField
component is not Encapsulated, being used in the same file as inApp.js
does not hide the component UserField from the outer world. To hide the component details we have to export the UserField component from a different file and then import the component in App.js file. - Unit testing for UserField is not possible, as the component is not isolated and is in the same file with App component.
2. Not using setState callback to update state correctly
Many times setState is used twice in the same function to update the state value, many developers forget to use the callback provided by the setState, which might not update the state with the correct value.
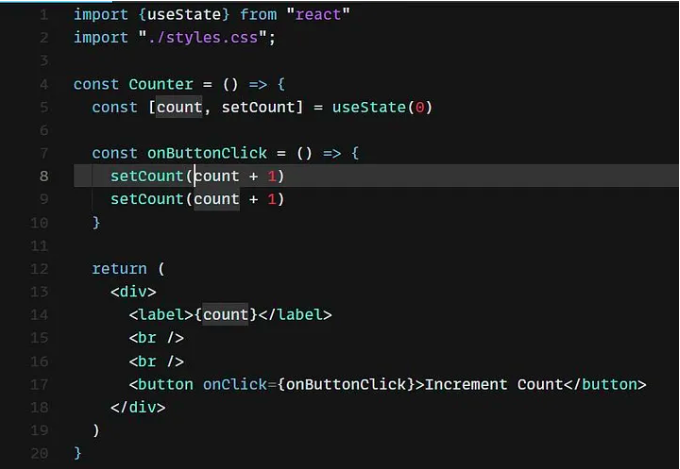
Mistake 2: State is not updated with correct value.
In the above code for Counter, after clicking on the button the count value will be increased only once though it should be incremented by 2. After clicking the button the count value will be 1 . This is because in case of the second setCount (line 8) the count value is still 0 and is not updated because in React, the state value is always after the execution of the function. That’s why even for the count in second setCount is 0.
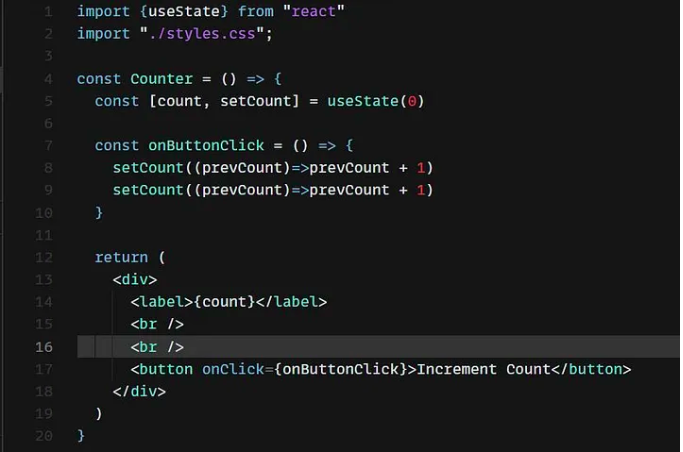
Mistake 2: Fixing the state value.
Using the callback provided by setState method can fix this issue. This is because now in case of the second setState, it will consider the current/updated value of count and then will increment the count value by 1. After fixing the code, when the button is clicked, the count is updated correctly. The count is now incremented by 2.
3. Invoking components with wrong syntax
Many times the components are invoked directly using JS functions which created many issues.
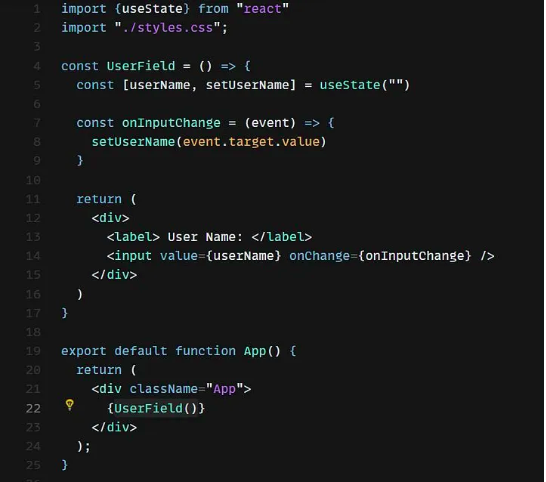
Mistake 3: Invoking components directly.
The above code looks fine and when executed, the working of the code will look fine though its having the following issues:
- By directly invoking the component, we are basically creating a custom hook of
UserField
and the code is re-rendered multiple times when the value is updated in the input field. - With the above code, not only the
UserField
component is re-rendered but also theApp
component is re-rendered when the state is updated in UserField component. This is because invoking the component directly, the component is now being treated as a custom hook and every time the state value in the component is updated, the HTML is returned causing re-rendering of App component as well.
To fix this, instead of directly invoking the component we have to call the component as an element <UserField />
.
Conclusion
In conclusion, React is a powerful and flexible library for building complex user interfaces. However, it’s easy to make mistakes when working with React. Some of the most common mistakes include not using state properly, invoking components with wrong syntax, not understanding the component lifecycle, and not optimizing performance.
Remember, React is just one part of a larger ecosystem of web development technologies. It’s important to understand how React fits into the bigger picture, and to be comfortable using other tools and frameworks as needed. By keeping these tips in mind, you can avoid common React mistakes and build better, more effective applications.
Visit deqode for more such tutorials and guides.